Python读取sqlite3数据库中的数据
## 1. 简介
- 从Python3.x版本开始,在标准库中已经**内置**了SQLlite3模块,它可以支持SQLite3数据库的访问和相关的数据库操作。在需要操作SQLite3数据库数据时,只须在程序中导入SQLite3模块即可。
## 1.1. 使用
### 1.1.1. 创建
代码
```
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('first.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'create table t_person(pno INTEGER PRIMARY KEYAUTOINCREMENT ,pname varchar(30) NOT NULL ,age INTEGER)'
try:
cur.execute(sql)
except Exception as e:
print(e)
print('创建表失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
```
- 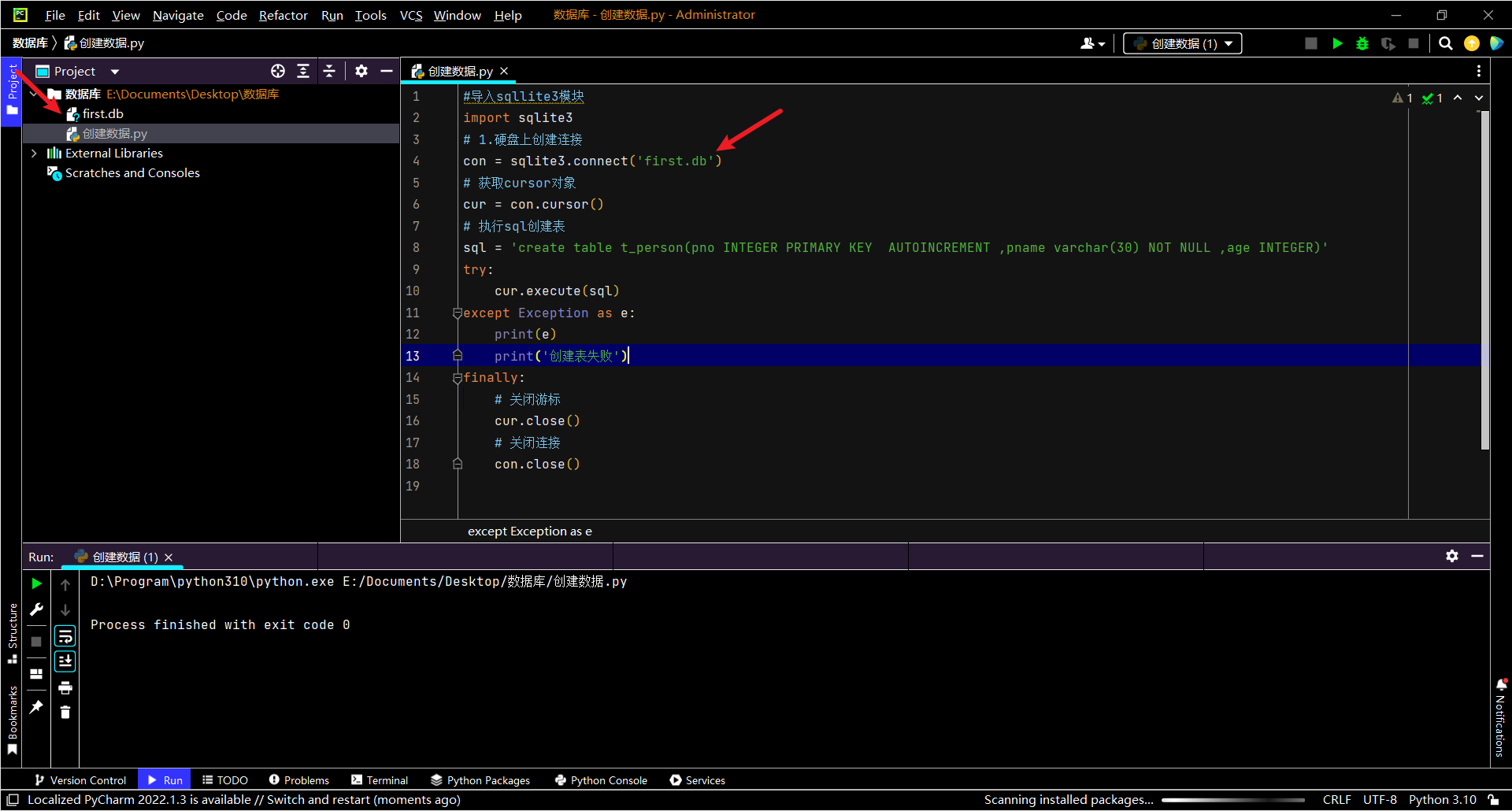
### 1.1.2. 插入
#### 1.1.2.1. 插入一条数据
```
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('first.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'insert into t_person(pname,age) values(?,?)'
try:
cur.execute(sql,('张三',23))
#提交事务
con.commit()
print('插入成功')
except Exception as e:
print(e)
print('插入失败')
con.rollback()
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
```
### 1.1.3. 查询
#### 1.1.3.1. 查询所有数据
- fetchall()查询所有数据
```
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('first.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'select * from t_person'
try:
cur.execute(sql)
# 获取所有数据
person_all = cur.fetchall()
# print(person_all)
# 遍历
for p in person_all:
print(p)
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
```
-
- 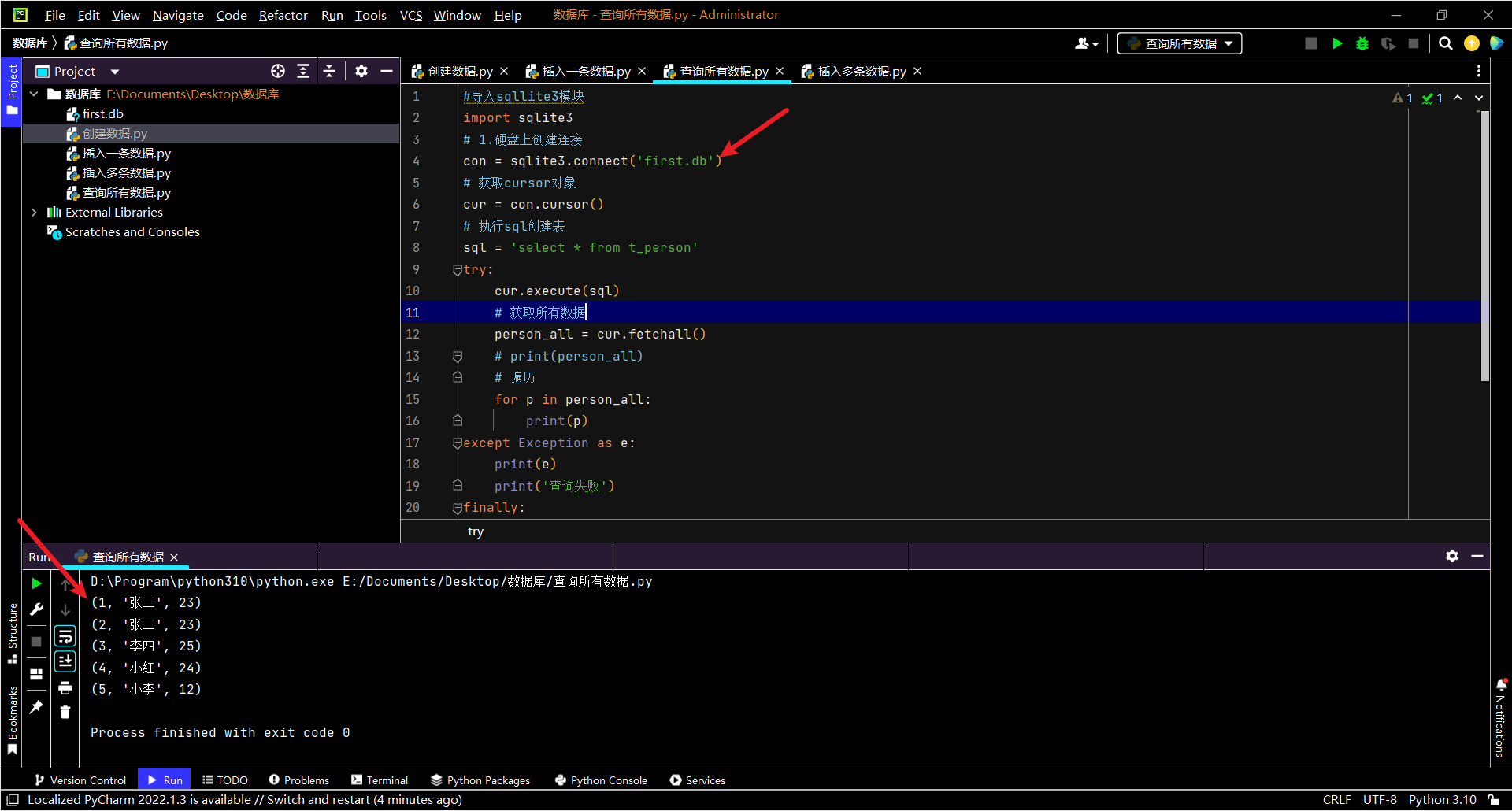
- #### 查询一条数据
- fetchone()查询一条数据
- 按顺序进行读取
```
#导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect('first.db')
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
sql = 'select * from t_person'
try:
cur.execute(sql)
# 获取一条数据
person = cur.fetchone()
print(person)
person = cur.fetchone()
print(person)
person = cur.fetchone()
print(person)
person = cur.fetchone()
print(person)
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
```
-
- 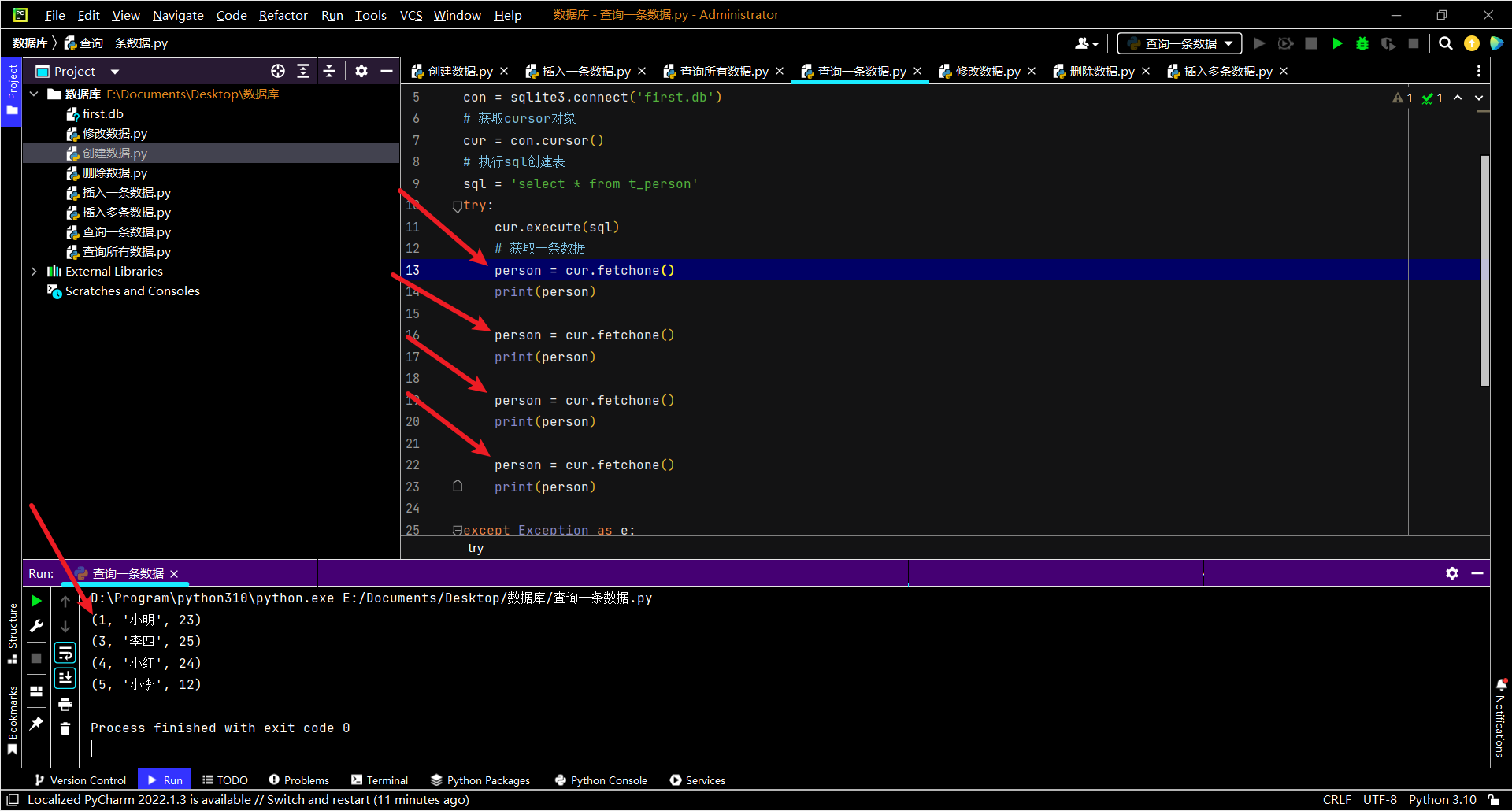
#### 1.1.3.2. 读取特定位置的数据
- 需要把读取的信息放到sql语句中
获得最新一条数据
- 代码
```
def sqlite3_get_last_data(db_path,sql):
# 导入sqllite3模块
import sqlite3
# 1.硬盘上创建连接
con = sqlite3.connect(db_path)
# 获取cursor对象
cur = con.cursor()
# 执行sql创建表
try:
cur.execute(sql)
# 获取所有数据
person_all = cur.fetchall()
last_data = person_all[-1]
# print(last_data)
# print("type(last_data):", type(last_data))
# print("last_data:", )
last_text = last_data
return last_text
except Exception as e:
print(e)
print('查询失败')
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
db_path = 'D:\MailMasterData\hengzhe19711121@163.com_1414\search.db'
sql = 'select * from Search_content'
last_text = sqlite3_get_last_data(db_path,sql)
print("last_text:", last_text)
```
- 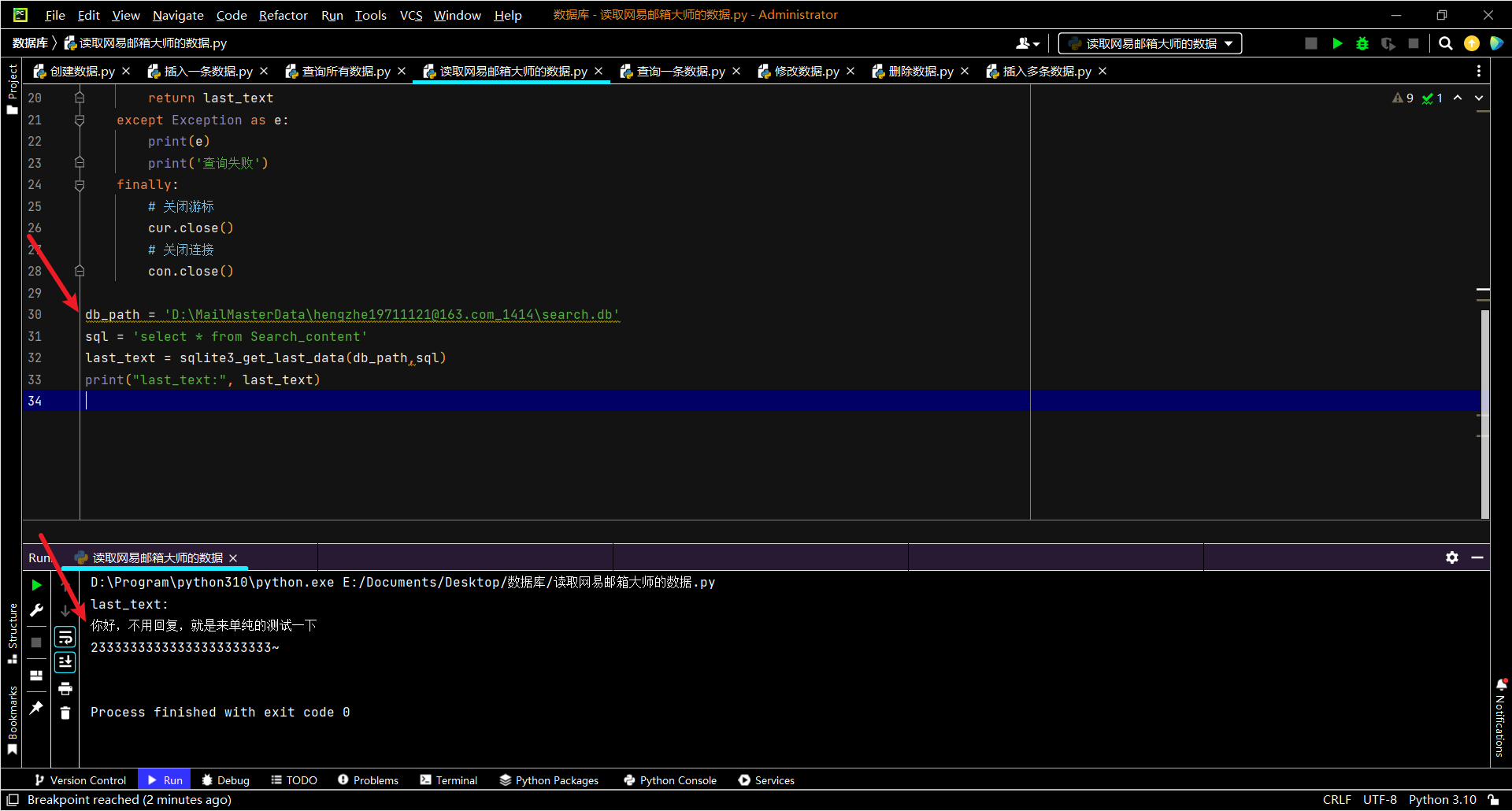
-
### 1.1.4. 修改数据
代码
```
#导入sqllite3模块
import sqlite3
#1.硬盘上创建连接
con=sqlite3.connect('first.db')
#获取cursor对象
cur=con.cursor()
try:
#执行sql创建表
update_sql = 'update t_person set pname=? where pno=?'
cur.execute(update_sql, ('小明', 1))
#提交事务
con.commit()
print('修改成功')
except Exception as e:
print(e)
print('修改失败')
con.rollback()
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
```
- 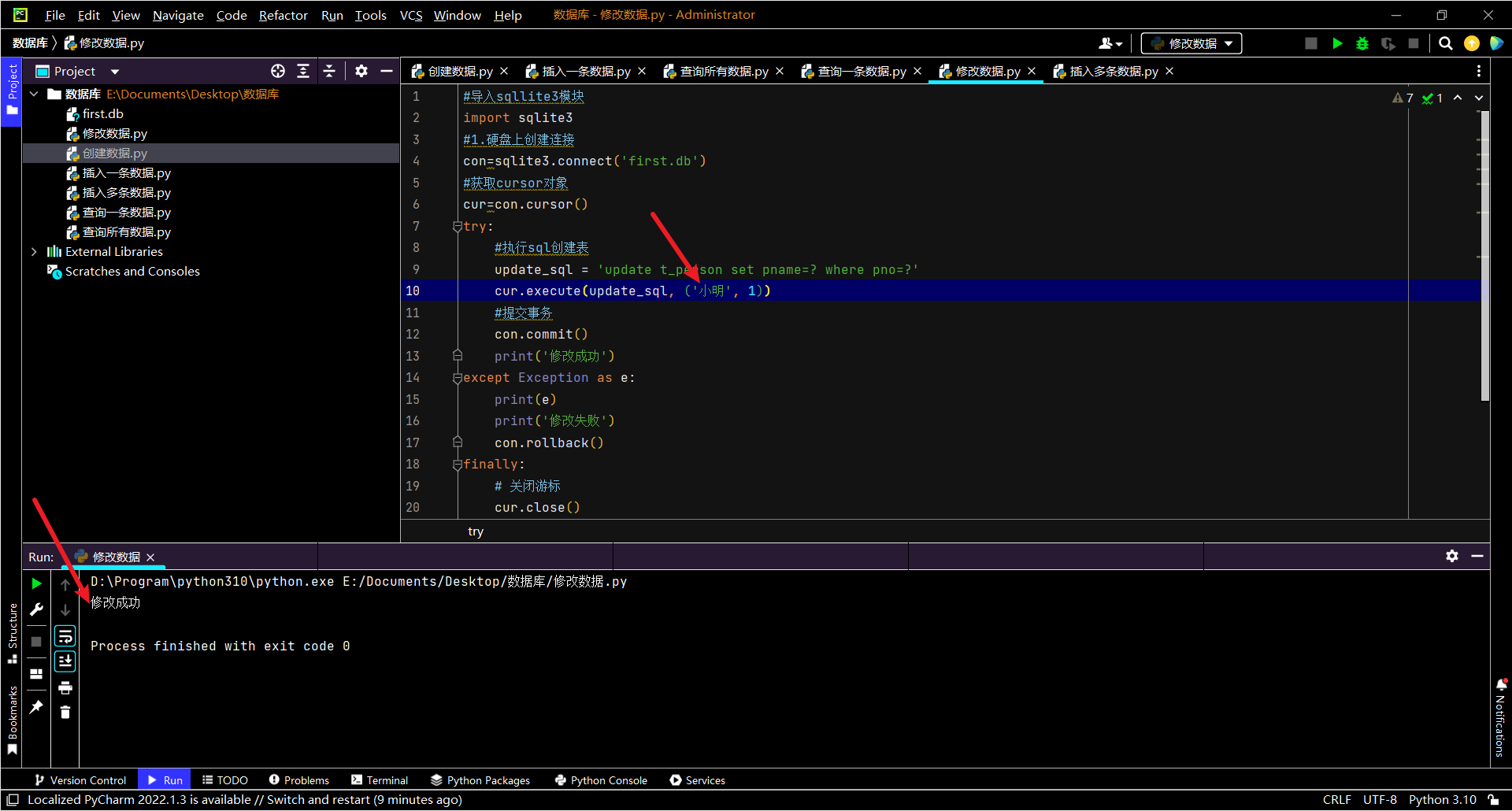
### 1.1.5. 删除数据
代码
```
#导入sqllite3模块
import sqlite3
#1.硬盘上创建连接
con=sqlite3.connect('first.db')
#获取cursor对象
cur=con.cursor()
#执行sql创建表
delete_sql = 'delete from t_person where pno=?'
try:
cur.execute(delete_sql, (2,))
#提交事务
con.commit()
print('删除成功')
except Exception as e:
print(e)
print('删除失败')
con.rollback()
finally:
# 关闭游标
cur.close()
# 关闭连接
con.close()
```
- 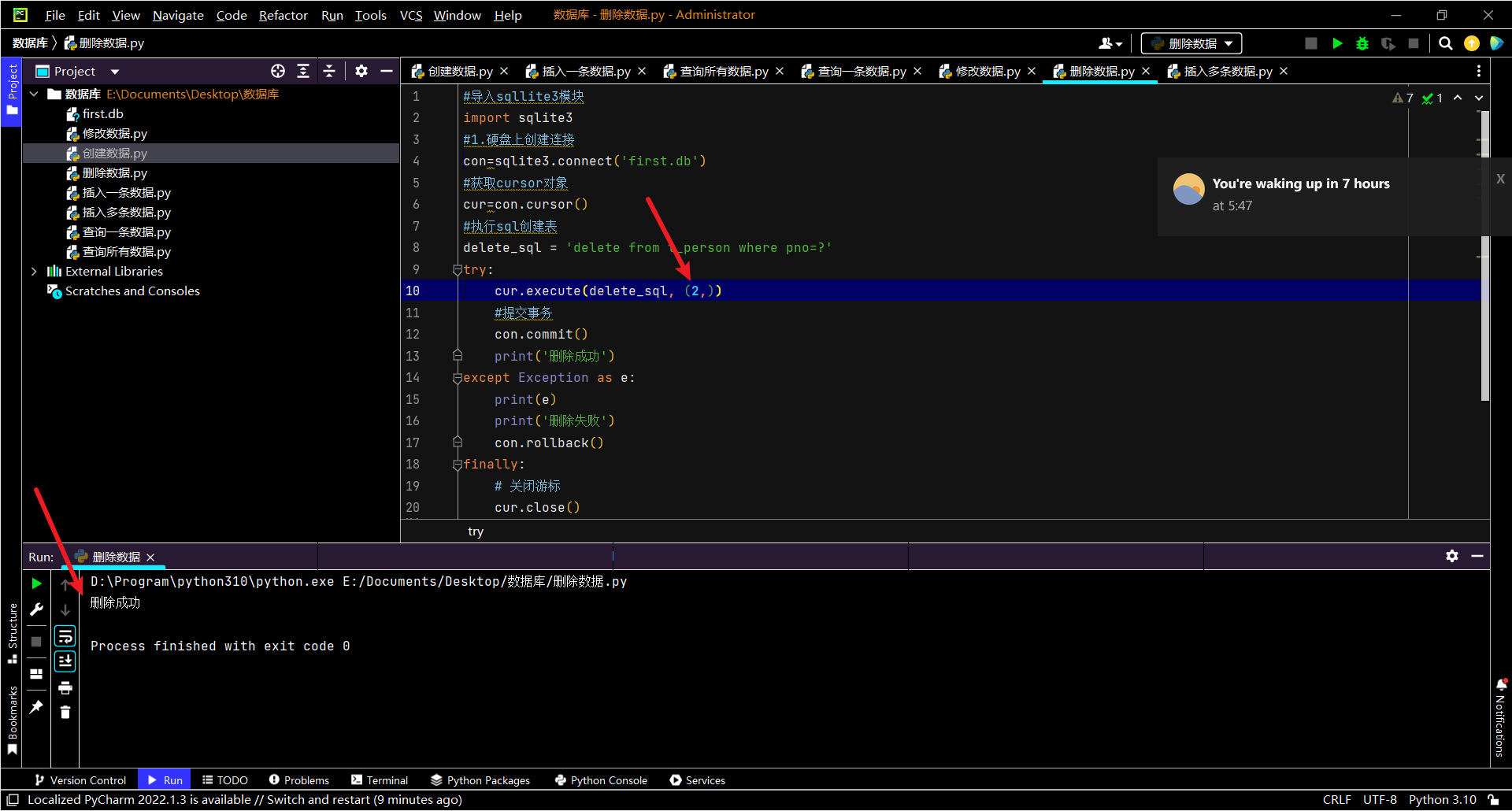 GMCN 发表于 2022-12-29 10:11
支持加密吗
默认的没有
需要安装 sqlcipher , 然后就可以了
from pysqlcipher3 import dbapi2 as sqlite3
....
cursor.execute("PRAGMA key='mypassword'")
python处理sqlite数据,大概150万行数据,读取到内存里会爆,只能用sql将处理放在sqlite内处理,但是效率低下,请问有没有办法加载在内存里处理? {:301_1002:}{:301_974:}{:301_973:}前排支持 谢谢锟斤拷锟斤拷 Python读取sqlite3 {:1_921:} 6666666666666666666 支持加密吗 感谢楼主分享,支持一下! 感谢分享,非常实用的库 感谢分享。 还不错,支持!!!
页:
[1]
2