前言
如果对Python完全不懂,请阅读我之前的贴文:Python笔记(从不懂到入门)补充版
(链接:https://www.52pojie.cn/thread-1225969-1-1.html )这篇文章跟我那篇文章相配合。此文不适合熟悉Python的人士,只适合刚刚学习Python的人。
1. 订立制作目标
做任何程序(生活也是如此)之前,必须做一个规划,就是说你想做什么、你想做到什么。
目标:
- 输入两个数字,一个是最低值,一个是最高值
- 随机生成一个数字
重复:
- 问他需不需要再生成或者关闭程序
-
重复运行第1-3的代码
2. 制作所需函数或模块
根据‘目标’,估算所需函数或者模块
- 输入两个数字,一个是最低值,一个是最高值 (变量、input函数、int数据类型)
- 随机生成一个数字 (random模块、print函数)
重复:
- 问他需不需要再生成或者关闭程序 (exit函数、if函数、input函数、变量、int数据类型)
- 重复运行第1-3的代码 (while函数)
3. 开始制作
1. 第一步(import模块)
由于Python经常把模块放在最前,所以首先要import(载入、输入)函数。
- 随机生成一个数字 (random模块、print函数)
- 问他需不需要再生成或者关闭程序 (exit函数、if函数、input函数、变量、int数据类型)
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
2. 第二步
- 输入两个数字,一个是最低值,一个是最高值 (变量、input函数、int数据类型)
我用‘low’代表最低值;‘high’代表最高值
备注:由于复杂程序需要用到很多变量,所以最好变量命名是跟该代码有关,以免混淆
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
3. 第三步
- 随机生成一个数字 (random模块、print函数)
random 函数格式
random.randint(最低值,最高值)
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
print(random.randint(low,high))
4. 第四步
- 问他需不需要再生成或者关闭程序 (exit函数、if函数、input函数、变量、int数据类型)
用‘ask’代表回答
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
print(random.randint(low,high))
ask=int(input('输入1代表继续,0代表结束程序,请输入:'))
if ask==1:
print('继续')
elif ask==0:
exit()
else:
print(输入有误,请重新输入。)
对了,如何弄最后那句重新输入?
这就要用到while函数了。
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
print(random.randint(low,high))
ask=int(input('输入1代表继续,0代表结束程序,请输入:'))
while ask==0 or 1:
if ask==1:
print('继续')
elif ask==0:
exit()
else:
print('输入有误,请重新输入。')
5. 第五步
- 重复运行第1-3的代码 (while函数)
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
while ask==1:
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
print(random.randint(low,high))
ask=int(input('输入1代表继续,0代表结束程序,请输入:'))
while ask==0 or 1:
if ask==1:
print('继续')
elif ask==0:
exit()
else:
print('输入有误,请重新输入。')
3. 运行并debug(解决错误)
第一次运行
运行截图:
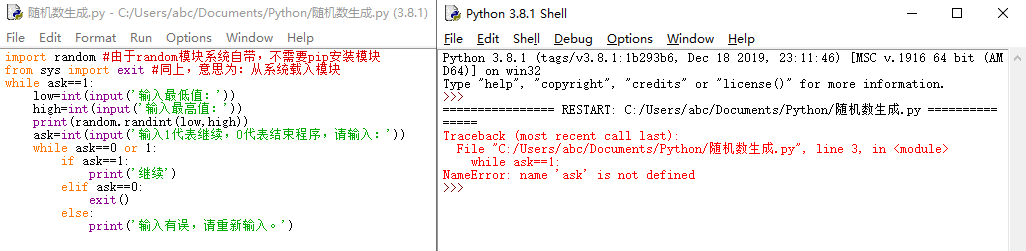
Bug 错误
Traceback (most recent call last):
File "C:/Users/abc/Documents/Python/随机数生成.py", line 3, in <module>
while ask==1:
NameError: name 'ask' is not defined
最重要的还是最后一句:未定义名称‘Ask’
debug
要在前面新增一句ask=1
完整代码:
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
ask=1
while ask==1:
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
print(random.randint(low,high))
ask=int(input('输入1代表继续,0代表结束程序,请输入:'))
while ask==0 or 1:
if ask==1:
print('继续')
elif ask==0:
exit()
else:
print('输入有误,请重新输入。')
第二次运行
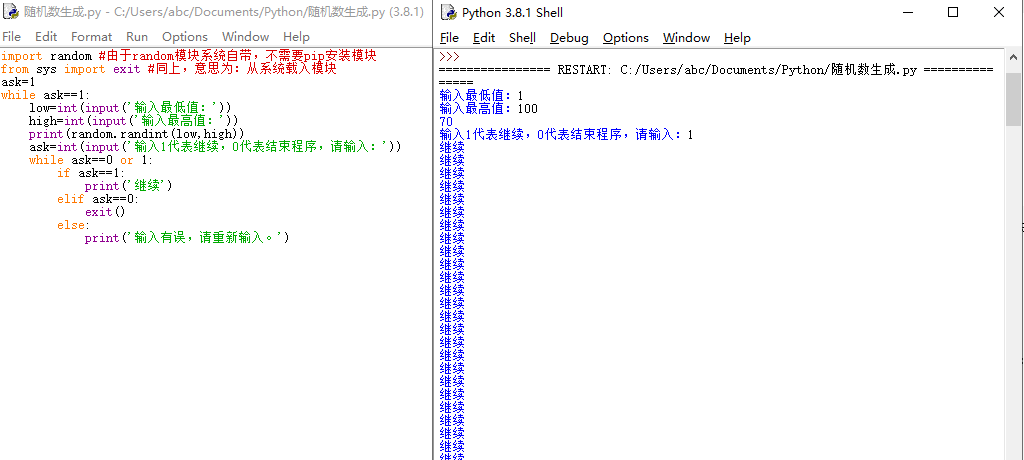
bug
看上图,可以见到再不停运行,如何解决?
debug
我们可以用break
放在print('继续')
后面。
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
ask=1
while ask==1:
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
print(random.randint(low,high))
ask=int(input('输入1代表继续,0代表结束程序,请输入:'))
while ask==0 or 1:
if ask==1:
print('继续')
break
elif ask==0:
exit()
else:
print('输入有误,请重新输入。')
第三次运行
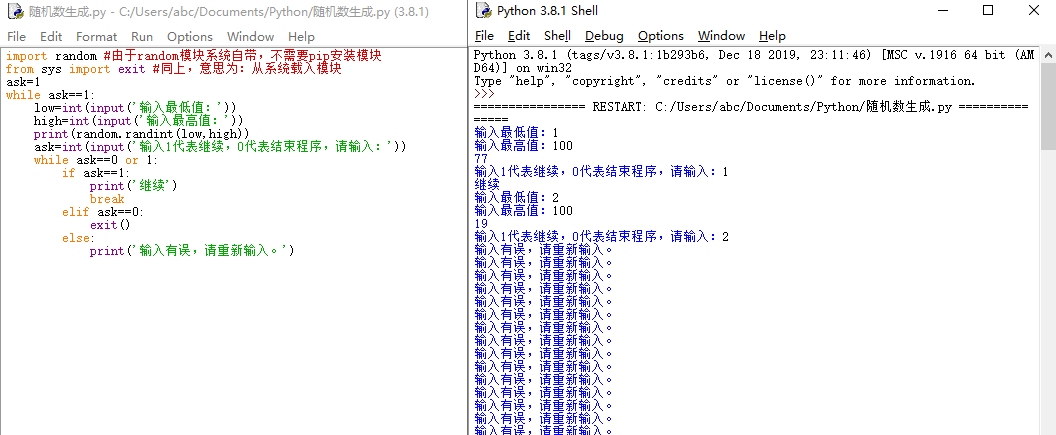
bug
问题如上,不多说,都是加break
debug
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
ask=1
while ask==1:
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
print(random.randint(low,high))
ask=int(input('输入1代表继续,0代表结束程序,请输入:'))
while ask==0 or 1:
if ask==1:
print('继续')
break
elif ask==0:
exit()
else:
print('输入有误,请重新输入。')
break
第四次运行
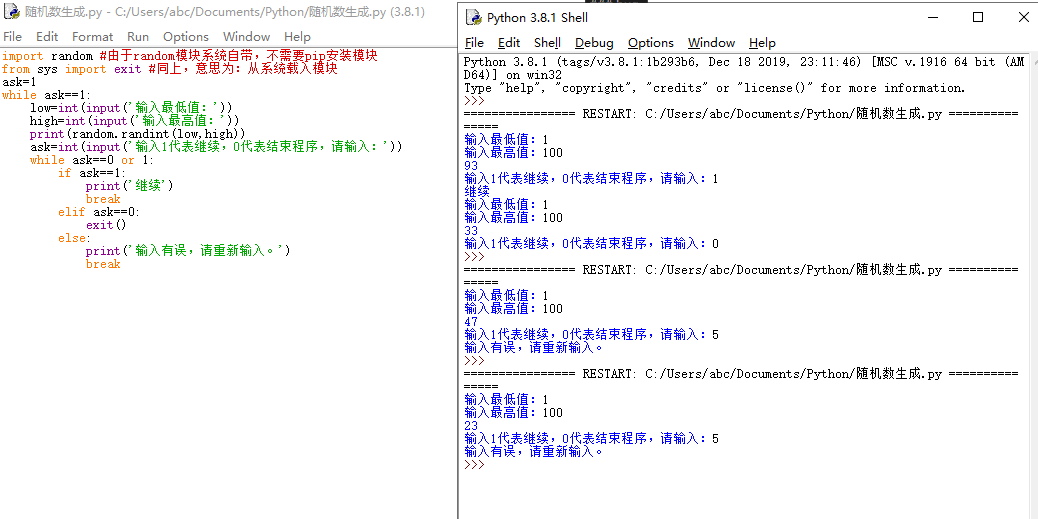
Bug
输入错误后,不能重新输入
- 原因:加了
break
debug
import random #由于random模块系统自带,不需要pip安装模块
from sys import exit #同上,意思为:从系统载入模块
ask=1
while ask==1:
low=int(input('输入最低值:'))
high=int(input('输入最高值:'))
print(random.randint(low,high))
ask=int(input('输入1代表继续,0代表结束程序,请输入:'))
while ask==0 or 1:
if ask==1:
print('继续')
break
elif ask==0:
exit()
else:
print('输入有误,请重新输入。')
ask=1
P.S. 这个方法不算最好,但是实在想不出更好的方法,欢迎大佬在评论区指导。
第五次运行
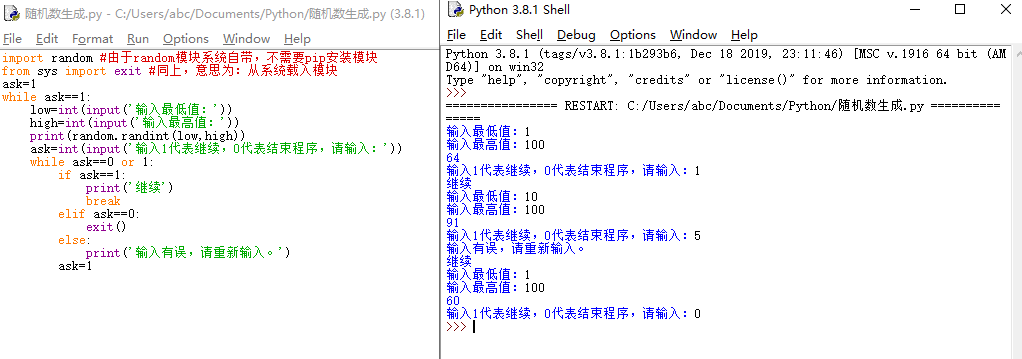
程序尚算成功。
后话
希望这个教程可以让新手慢慢学会制作一个程序。如有疑问,欢迎在评论区留言。如果觉得这篇文章对你有用,欢迎评分