自学Java-0基础到框架(JDK8)-day06
数组
声明
两种声明变量方式
1. 类型[] 变量名;
2. 类型 变量名[];
例子:
1. int[] numArray;
2. int numTest[];
创建
示例:
1. int[] a = new int[10];
// 10代表数组的长度
2. int[] b = {1,2,3,4,5,6,7}
因为数组元素大小和类型都是确定的,可以使用for循环或者是之前提到的加强for循环对数组进行处理!
这里对加强for循环进行一个复习。
示例:
public class ArrayTest {
public static void main(String[] args) {
int[] a = {1, 2, 3, 4, 5, 6, 7, 8, 9};
for (int num : a) {
System.out.println(num);
}
}
}
输出:
1
2
3
4
5
6
7
8
9
作为参数
同样数组可以作为传入方法中的参数
Java 基本数据类型传递参数时是值传递 ;引用类型传递参数时是引用传递 。数组是引用传递
示例:
public class ArrayTest {
public static void main(String[] args) {
int[] a = {1, 2, 3, 4, 5, 6, 7, 8, 9};
printArray(a);
}
public static void printArray(int[] array) {
for (int i = 0; i < array.length; i++) {
System.out.println(array[i]);
}
}
}
Out:
1
2
3
4
5
6
7
8
9
作为返回值
示例:
public class ArrayTest {
public static void main(String[] args) {
int[] c = returnArray(2, 11);
for(int i:c){
System.out.println(i);
}
}
public static int[] returnArray(int a, int b) {
int arrayLength = b - a + 1;
int[] array = new int[arrayLength];
for (int i = a; i <= b; i++) {
array[i - a] = i;
}
return array;
}
Out:
2
3
4
5
6
7
8
9
10
11
多维数组
// 创建一个二维数组,并随机赋值
int[][] array = new int[5][5];
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[i].length; j++) {
array[i][j] = (int) (Math.random() * 100);
}
}
// 打印二维数组
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[i].length; j++) {
System.out.print(array[i][j] + "\t");
}
System.out.println();
}
Out:
66 96 37 67 32
40 18 36 44 31
34 20 27 79 20
78 43 1 69 66
65 46 99 77 52
Arrays 类
java.util.Arrays 类能方便地操作数组,它提供的所有方法都是静态的。
具有以下功能:
- 给数组赋值:通过 fill 方法。
- 对数组排序:通过 sort 方法,按升序。
- 比较数组:通过 equals 方法比较数组中元素值是否相等。
- 查找数组元素:通过 binarySearch 方法能对排序好的数组进行二分查找法操作。
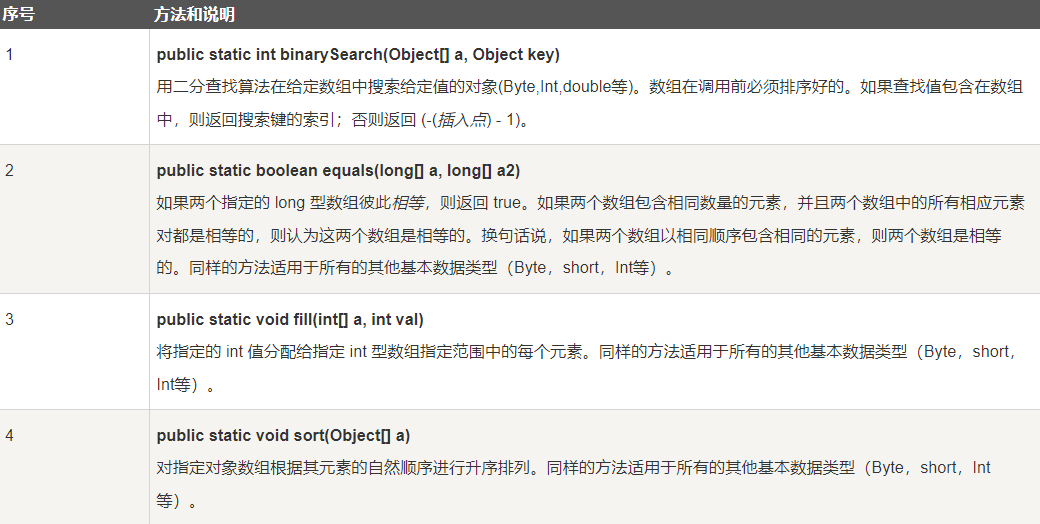
正则表达式
java.util.regex 包主要包括以下三个类:
-
Pattern 类:
pattern 对象是一个正则表达式的编译表示。Pattern 类没有公共构造方法。要创建一个 Pattern 对象,你必须首先调用其公共静态编译方法,它返回一个 Pattern 对象。该方法接受一个正则表达式作为它的第一个参数。
-
Matcher 类:
Matcher 对象是对输入字符串进行解释和匹配操作的引擎。与Pattern 类一样,Matcher 也没有公共构造方法。你需要调用 Pattern 对象的 matcher 方法来获得一个 Matcher 对象。
-
PatternSyntaxException:
PatternSyntaxException 是一个非强制异常类,它表示一个正则表达式模式中的语法错误。
示例:
public class RegexTest {
public static void main(String[] args) {
String str = "This is a test string";
String regex = ".*test.*";
System.out.println(str.matches(regex));
}
}
//Out: true
import java.util.regex.*;
public class RegexTest {
public static void main(String[] args) {
String str = "This is a test string";
String regex = ".*test.*";
boolean isMatch = Pattern.matches(regex, str);
System.out.println(isMatch);
}
}
//Out:true
突然有点事,明天继续学~~~